Hi all,
One of our clients ran into an issue which rendered her unable to edit/check out documents from MOSS. Whenever she would try to open a file, the following error message would occur:
lol @ the "Was this information helpful?". Of course it's not! Experience has proven me that often these type of err msgs are misleading as you'll find out in a moment. At first I thought it was a user rights permission issue on that document library but other people with the same rights had no problems editing. This is an indication that the problem might reside at the client and could be related to corrupted cached files. So next thing I did was check the Microsoft Office Upload Center for any pending files. Nothing.
Then I found out that some necessary add-on components were missing from her IE, in particular the 'SharePoint OpenDocuments Class'. This probably happened after an update. The way I solved it is as follows:
1. Go to 'Control Panel' -> 'Programs' -> 'Programs and Features'
2. Find the version of Office you're using, in my case it was 'Microsoft Office Professional Plus 2010'
3. Click the 'Change' button
4. From the menu, choose the 'Repair' option and click 'Continue'
Now this might take a minute of 10-15 and afterwards, it will prompt you to reboot. Do so and as soon as you're logged in again, check if the SharePoint addons are present in IE by:
1. Opening IE
2. Click 'Tools' (or ALT+X)
3. Click 'Manage Add-ons'
4. Change the 'Show' dropdown to 'All add-ons'
5. Sort by Name and verify if you see the various SharePoint add-ons, especially the 'SharePoint OpenDocuments Class'
Ciao!
Keywords
administration
(2)
AJAX
(1)
arrays
(1)
asp
(2)
ASP.NET
(2)
assembly
(2)
automation
(1)
BCS
(1)
bindings
(1)
C#
(10)
cell click
(1)
cell value
(1)
clientside
(2)
connection string
(1)
content types
(2)
CSS
(1)
csv
(1)
datagrid
(2)
delegates
(1)
design patterns
(1)
Dialog
(4)
Dictionary
(1)
domain controller
(1)
domain member
(1)
dropdown list
(2)
endpoints
(1)
enum
(1)
error
(1)
event handlers
(1)
Excel
(1)
exporting
(1)
feature event receiver
(2)
File
(1)
formatting code
(1)
forms
(2)
Function
(1)
gac
(1)
hotfolder
(1)
HTML
(2)
inheritance
(1)
javascript
(4)
keyvalue
(1)
layoutspagebase
(1)
LINQ
(2)
lists
(3)
machine account password
(1)
moss
(1)
namespaces
(1)
ObjectDataSource
(1)
objects
(1)
office
(1)
OleDbConnection
(1)
pairs
(1)
permissions
(1)
pivot
(1)
postback
(1)
powershell
(2)
radconfirm
(1)
registry
(1)
resources
(1)
role definitions
(1)
rowclick
(1)
serialization
(2)
serverside
(4)
sharepoint
(15)
site columns
(3)
snapshot
(1)
SPList
(2)
sql
(1)
sql server
(2)
string
(1)
t-sql
(2)
Telerik
(3)
TFS
(1)
Timer
(1)
timerjob
(1)
transpose
(1)
txt
(2)
update
(1)
VBA
(2)
ViewState
(2)
Visual Studio
(1)
web.config
(1)
webparts
(4)
webservices
(1)
XDocument
(1)
xml
(2)
Wednesday, November 20, 2013
Wednesday, September 18, 2013
Failed trust relationship between workstation and primary domain
Hi,
This morning I restored a snapshot on my virtual machine which I took about six weeks ago. When I tried
to log on using my user account which is in the company domain, Windows confronted me with the following
login error:
"The trust relationship between this workstation and the primary domain failed".
Using the local administrator's account I was able to log on. So let's break down what actually happened
here.
The machine has a default setting in the registry which determines how often your machine will try to change its machine account password. This password is being used to secure a safe communication channel between the domain member and domain controller. In this channel information about authentication and authorization decisions are being transmitted, thus must be handled carefully.
So, what happened here is that after I took the snapshot, the machine account password was changed (this is done by the Netlogon service) and the new password had also been updated in the domain controller so everything was in synch. By restoring the snapshot, I got back the old password and this caused a mismatch between the domain member and the domain controller.
NOTE: This can be solved by removing the computer (or VM in this case) from the domain and then re-adding it.
Now, to prevent this from happening again, do the following:
1. Press Windows Logo Key + R
2. Type 'gpedit.msc' in the textbox and press Enter
3. Expand 'Computer Configuration' -> 'Windows Settings' -> 'Security Settings' -> 'Local Policies' -> 'Security Options'
4. Search for the policy called: 'Domain member: Disable machine account password changes'
5. Double-click it, change the setting to 'Enabled' and click 'OK'
This is obviously not the safest option to choose security-wise, however, in my case I'm dealing with a development machine so
I am cool with this.
Alternatively you can also choose to keep this option disabled and rather change the 'Domain member: Maximum machine account password age' and set it to a value of which you think is more suitable.
Hope this helps.
Later.
This morning I restored a snapshot on my virtual machine which I took about six weeks ago. When I tried
to log on using my user account which is in the company domain, Windows confronted me with the following
login error:
"The trust relationship between this workstation and the primary domain failed".
Using the local administrator's account I was able to log on. So let's break down what actually happened
here.
The machine has a default setting in the registry which determines how often your machine will try to change its machine account password. This password is being used to secure a safe communication channel between the domain member and domain controller. In this channel information about authentication and authorization decisions are being transmitted, thus must be handled carefully.
So, what happened here is that after I took the snapshot, the machine account password was changed (this is done by the Netlogon service) and the new password had also been updated in the domain controller so everything was in synch. By restoring the snapshot, I got back the old password and this caused a mismatch between the domain member and the domain controller.
NOTE: This can be solved by removing the computer (or VM in this case) from the domain and then re-adding it.
Now, to prevent this from happening again, do the following:
1. Press Windows Logo Key + R
2. Type 'gpedit.msc' in the textbox and press Enter
3. Expand 'Computer Configuration' -> 'Windows Settings' -> 'Security Settings' -> 'Local Policies' -> 'Security Options'
4. Search for the policy called: 'Domain member: Disable machine account password changes'
5. Double-click it, change the setting to 'Enabled' and click 'OK'
This is obviously not the safest option to choose security-wise, however, in my case I'm dealing with a development machine so
I am cool with this.
Alternatively you can also choose to keep this option disabled and rather change the 'Domain member: Maximum machine account password age' and set it to a value of which you think is more suitable.
Hope this helps.
Later.
Thursday, August 29, 2013
Convert an Excel sheet to a .CSV file using C#
Hi guys,
Before going on vacation, I wanted to share a handy little piece of code to convert an Excel sheet to a .csv file.
Before going on vacation, I wanted to share a handy little piece of code to convert an Excel sheet to a .csv file.
static void ConvertXlsToCsv(string sheetTitle, string inputFile, string outputFile) { try { string strConn = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" + inputFile + ";Extended Properties=\"Excel 12.0;HDR=No;IMEX=1\";"; var output = new DataSet(); using (var conn = new OleDbConnection(strConn)) { conn.Open(); var schemaTable = conn.GetOleDbSchemaTable(OleDbSchemaGuid.Tables, new object[] { null, null, sheetTitle + "$", "TABLE" }); var cmd = new OleDbCommand("SELECT * FROM [" + sheetTitle + "$]", conn); cmd.CommandType = CommandType.Text; OleDbDataAdapter adapter = new OleDbDataAdapter(cmd); adapter.Fill(output, sheetTitle + "$"); var dt = new DataTable(); adapter.Fill(dt); using (var sw = new StreamWriter(outputFile)) { for (int y = 0; y < dt.Rows.Count; y++) { var strRow = ""; for (int x = 0; x < dt.Columns.Count; x++) { strRow += "\"" + dt.Rows[y][x].ToString() + "\","; } sw.WriteLine(strRow); } } } } catch (Exception exc) { // Exception handling logic } }
Wednesday, July 17, 2013
Sudden "Unable to display this Web Part. To troubleshoot the..." error
Hi guys,
Yesterday I was unpleasantly surprised with an error trying to view a list:
"Unable to display this Web Part. To troubleshoot the problem, open this Web page in a Microsoft SharePoint Foundation-compatible HTML editor such as Microsoft SharePoint Designer. If the problem persists, contact your Web server administrator.
Correlation ID: ....."
After drilling down the log, some investigation, coffee and googling, I found out that this problem is caused by a Windows update, to be more specific; KB2844286. It's a security update but it renders your list (and listview webparts) inaccessible if you've got any custom XSLT going on in there. Uninstalling the update and performing an IISreset on the WFE solved the issue.
Yesterday I was unpleasantly surprised with an error trying to view a list:
"Unable to display this Web Part. To troubleshoot the problem, open this Web page in a Microsoft SharePoint Foundation-compatible HTML editor such as Microsoft SharePoint Designer. If the problem persists, contact your Web server administrator.
Correlation ID: ....."
After drilling down the log, some investigation, coffee and googling, I found out that this problem is caused by a Windows update, to be more specific; KB2844286. It's a security update but it renders your list (and listview webparts) inaccessible if you've got any custom XSLT going on in there. Uninstalling the update and performing an IISreset on the WFE solved the issue.
Labels:
administration,
error,
lists,
sharepoint,
update,
webparts
Thursday, July 4, 2013
Implementing a simple custom Timer Job in SharePoint 2010
Simple implementation of an SPTimerJob which moves all files in a specified folder to another, prefixing the filenames with a timestamp. The interval is set to every minute.
1. Create a new empty SharePoint 2010 project
2. Create the following class (make sure the folders specified have been created:
4. Add an event receiver to it and define the activated/deactivated methods as follows:
6. Deploy and see the magic happen
1. Create a new empty SharePoint 2010 project
2. Create the following class (make sure the folders specified have been created:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Microsoft.SharePoint.Administration; using System.IO; namespace HotFolderJob { public class FileMoverJob : SPJobDefinition { #region Constructors //Constructor public FileMoverJob() : base() { } //Constructor public FileMoverJob(string name, SPWebApplication webApp) : base(name, webApp, null, SPJobLockType.ContentDatabase) { this.Title = "Hotfolder Timer Job"; } #endregion Constructors #region Methods - Overridden public override void Execute(Guid targetInstanceId) { const string sourcePath = @"C:\TimerJob\DropFolder"; const string targetPath = @"C:\TimerJob\TargetFolder"; if (System.IO.Directory.Exists(sourcePath)) { string[] fullPathFiles = System.IO.Directory.GetFiles(sourcePath); foreach (string fullPathfile in fullPathFiles) { var originalFileName = Path.GetFileName(fullPathfile); var destFile = Path.Combine(targetPath, (string.Format("{0:yyMMdd_Hmmss}_", DateTime.Now) + originalFileName)); File.Move(fullPathfile, destFile); //File.Copy(fullPathfile, destFile); } } } #endregion Methods - Overridden } }3. Add a feature, name it as you please
4. Add an event receiver to it and define the activated/deactivated methods as follows:
using System; using System.Runtime.InteropServices; using System.Security.Permissions; using Microsoft.SharePoint; using Microsoft.SharePoint.Security; using Microsoft.SharePoint.Administration; namespace HotFolderJob.Features.HotfolderFeature { [Guid("a2bb921d-dda5-4f0c-923c-da8004a4b09a")] public class HotfolderFeatureEventReceiver : SPFeatureReceiver { const string JOB_NAME = "HotFolderJob"; public override void FeatureActivated(SPFeatureReceiverProperties properties) { SPSite site = properties.Feature.Parent as SPSite; foreach (SPJobDefinition job in site.WebApplication.JobDefinitions) { if (job.Name == JOB_NAME) job.Delete(); } FileMoverJob fileMoverJob = new FileMoverJob(JOB_NAME, site.WebApplication); SPMinuteSchedule schedule = new SPMinuteSchedule(); schedule.BeginSecond = 0; schedule.EndSecond = 59; schedule.Interval = 1; fileMoverJob.Schedule = schedule; fileMoverJob.Update(); } public override void FeatureDeactivating(SPFeatureReceiverProperties properties) { SPSite site = properties.Feature.Parent as SPSite; foreach (SPJobDefinition job in site.WebApplication.JobDefinitions) { if (job.Name == JOB_NAME) job.Delete(); } } //public override void FeatureInstalled(SPFeatureReceiverProperties properties) //{ //} //public override void FeatureUninstalling(SPFeatureReceiverProperties properties) //{ //} //public override void FeatureUpgrading(SPFeatureReceiverProperties properties, string upgradeActionName, System.Collections.Generic.IDictionary<string, string> parameters) //{ //} } }5. Set the feature scope to Site (applies for this example)
6. Deploy and see the magic happen
Thursday, May 30, 2013
How to determine if an assembly is compiled as 32-bit or 64-bit
1. Go to VS Command Prompt
2. Execute the following: "dumpbin /headers mydll.dll" (of course replacing 'mydll.dll' with your assembly filename
3. Check the FILE HEADER VALUES and look for the value behind machine (x64 = 64-bit and x86 = 32-bit)
2. Execute the following: "dumpbin /headers mydll.dll" (of course replacing 'mydll.dll' with your assembly filename
3. Check the FILE HEADER VALUES and look for the value behind machine (x64 = 64-bit and x86 = 32-bit)
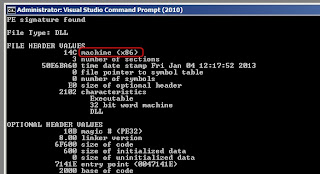
Wednesday, April 17, 2013
XMLNodeReader problem: The specified type was not recognized
// Solves the "The specified type was not recognized: name='##', namespace='##', at ..." // Came across this when trying to serialize an object of a custom type // It is caused due to a missing namespace reference (for some reason, the LookupNamespace method in the reader // does not return a string from the NameTable) public class XmlNodeReader2 : XmlNodeReader { public XmlNodeReader2(XmlNode node) : base(node) { } public override string LookupNamespace(string prefix) { return NameTable.Add(base.LookupNamespace(prefix)); } }
source:http://coderstuff.blogspot.nl/2005/10/xmlserializer-bug-when-xsitype-is-used.html
Tuesday, February 19, 2013
Retrieving a resource key in a custom LayoutsPageBase page used in SP2010
1. Create the following property in your aspx.cs file:
2. Create the following method which will return the requested value stored in the .resx file by looking up the resource key
3. Good to go. Say you would need to populate a label in the Page_Load event with a string stored in the resources file with a key called 'MyLabelText'; you can then achieve this as follows:
protected Type ResourceType { get { return typeof(MyResources); // replace 'MyResources' with your own resources file } }
2. Create the following method which will return the requested value stored in the .resx file by looking up the resource key
protected string GetResourceString(Type resourceType, string resourceKey) { return SPUtility.GetLocalizedString(string.Format("$Resources:{0}, {1}", resourceType.Name, resourceKey), resourceType.FullName, getLanguage); }
3. Good to go. Say you would need to populate a label in the Page_Load event with a string stored in the resources file with a key called 'MyLabelText'; you can then achieve this as follows:
protected void Page_Load(object sender, EventArgs e) { lblMyLabel.Text = GetSourceString(ResourceType, "MyLabelText"); }
Thursday, February 14, 2013
How to refresh parent page after closing a SP.UI.ModalDialog
var options = {
url:'/_layouts/YourAspx/YourPage.aspx',
title: 'Your Application Page Title',
allowMaximize: false,
showClose: true,
width: 700,
height: 260,
dialogReturnValueCallback: RefreshOnDialogClose
};
SP.UI.ModalDialog.showModalDialog(options);
Key here is the callback function; set it to RefreshOnDialogClose. Dead simple ;-)
url:'/_layouts/YourAspx/YourPage.aspx',
title: 'Your Application Page Title',
allowMaximize: false,
showClose: true,
width: 700,
height: 260,
dialogReturnValueCallback: RefreshOnDialogClose
};
SP.UI.ModalDialog.showModalDialog(options);
Key here is the callback function; set it to RefreshOnDialogClose. Dead simple ;-)
Subscribe to:
Posts (Atom)